What Is Destructor? A Comprehensive Guide To Understanding Destructors In Programming
So, here we are, diving deep into the world of destructors. If you're reading this, chances are you're either a coding enthusiast, a developer looking to sharpen your skills, or just plain curious about what destructors are all about. Let me tell ya, destructors are like the unsung heroes of programming. They’re the behind-the-scenes cleanup crew that makes sure your code runs smoothly without leaving a mess. But hey, let's not get ahead of ourselves. Before we jump into the nitty-gritty, let’s first break it down. Destructor is a special method in object-oriented programming that’s automatically called when an object is destroyed or goes out of scope.
Now, why should you care? Well, imagine building a house but never cleaning up after yourself. You’d end up with a pile of bricks, tools, and debris everywhere. Same goes for programming. If you don’t manage resources properly, you could end up with memory leaks, performance issues, and all sorts of chaos. That’s where destructors come in. They ensure that resources are released properly, preventing your program from turning into a disaster zone.
In this article, we’ll explore destructors in detail, covering everything from their purpose to practical use cases. Whether you’re a beginner or an experienced programmer, there’s something here for everyone. So, buckle up, and let’s get started!
Read also:Unlocking The Enigma Who Is Jhorany And Why Should You Care
Table of Contents
- What is Destructor?
- History of Destructor
- How Does Destructor Work?
- Types of Destructors
- Common Use Cases
- Benefits of Using Destructor
- Best Practices
- Common Mistakes to Avoid
- Real-World Examples
- Conclusion
What is Destructor?
Alright, let’s start with the basics. A destructor is essentially a method in programming that’s invoked automatically when an object is destroyed. Think of it like a cleanup crew that comes in after a party to tidy up the mess. In programming terms, the "mess" could be anything from allocated memory to open files or network connections. Destructors ensure that all these resources are properly released, preventing any potential issues down the line.
Now, here’s the thing: destructors are typically used in object-oriented programming languages like C++, C#, and Java. They’re especially important in languages where you have to manage memory manually, like C++. In languages with automatic garbage collection, destructors still play a role, but they’re more about releasing unmanaged resources rather than memory management.
Why Do We Need Destructors?
Let’s talk about the importance of destructors. Without them, your program could end up consuming more resources than necessary, leading to performance bottlenecks. For example, if you open a file but forget to close it, the file remains locked, and other processes can’t access it. Destructors ensure that such issues don’t occur by automatically closing the file when the object is destroyed.
History of Destructor
So, where did destructors come from? The concept of destructors was first introduced in the C++ programming language back in the early 1980s by Bjarne Stroustrup. The idea was simple yet powerful: provide a way to clean up resources automatically when an object is no longer needed. Since then, the concept has been adopted by other programming languages, each implementing it in its own way.
Over the years, destructors have evolved to become an essential part of modern programming practices. They’ve been refined to handle more complex scenarios, such as managing resources in multi-threaded environments or dealing with exceptions.
How Does Destructor Work?
Let’s dive into the mechanics of destructors. When an object is created, a constructor is called to initialize it. Similarly, when the object is destroyed, the destructor is invoked to clean up. The process is automatic, meaning you don’t have to manually call the destructor. It happens behind the scenes, ensuring that your program remains efficient and bug-free.
Read also:Joey Ray The Rising Star In The Music Scene You Need To Know
Key Features of Destructors
- Automatic Invocation: Destructors are called automatically when an object goes out of scope or is explicitly deleted.
- Resource Management: They handle the release of resources like memory, file handles, and database connections.
- Overloading Not Allowed: Unlike constructors, destructors cannot be overloaded. There can only be one destructor per class.
Types of Destructors
Now, here’s where things get interesting. Depending on the programming language and the context, destructors can take different forms. Let’s break them down:
1. Normal Destructor
This is the most common type of destructor. It’s automatically called when an object is destroyed. For example, in C++, the destructor is defined with a tilde (~) followed by the class name. Here’s how it looks:
~ClassName()
2. Virtual Destructor
Virtual destructors are used in polymorphic classes to ensure that the correct destructor is called when deleting an object through a base class pointer. This is crucial in preventing memory leaks and ensuring proper cleanup.
Common Use Cases
So, what are some practical scenarios where destructors are used? Let’s explore a few:
- Closing Files: Ensuring that files are closed properly after use.
- Releasing Memory: Freeing dynamically allocated memory to prevent memory leaks.
- Disconnecting from Databases: Closing database connections when they’re no longer needed.
- Releasing Locks: Unlocking mutexes or other synchronization objects in multi-threaded applications.
Benefits of Using Destructor
Here’s why destructors are worth your time:
- Automatic Resource Management: Reduces the risk of forgetting to release resources manually.
- Improved Performance: Prevents memory leaks and ensures efficient use of system resources.
- Code Simplicity: Eliminates the need for explicit cleanup code, making your code cleaner and easier to maintain.
Best Practices
Now that you know the importance of destructors, here are some tips to help you use them effectively:
- Avoid Throwing Exceptions: Destructors should not throw exceptions, as this can lead to undefined behavior.
- Keep It Simple: Avoid complex logic in destructors. They should focus solely on resource cleanup.
- Use Virtual Destructors in Polymorphic Classes: This ensures proper cleanup when deleting objects through base class pointers.
Common Mistakes to Avoid
Even the best programmers make mistakes, and destructors are no exception. Here are a few pitfalls to watch out for:
- Forgetting to Release Resources: This can lead to memory leaks and other resource-related issues.
- Throwing Exceptions: As mentioned earlier, destructors should not throw exceptions. Always handle errors gracefully.
- Not Using Virtual Destructors: This can cause improper cleanup in polymorphic classes, leading to undefined behavior.
Real-World Examples
Let’s look at a couple of real-world examples to see destructors in action:
Example 1: File Handling in C++
Suppose you’re working on a program that reads data from a file. You can use a destructor to ensure that the file is closed properly, even if an exception occurs:
class FileReader { public: FileReader(const std::string& filename) : file(filename) {} ~FileReader() { if (file.is_open()) file.close(); } private: std::ifstream file; };
Example 2: Database Connection Management
In a web application, you might need to manage database connections. A destructor can help ensure that connections are closed properly:
class DatabaseConnection { public: DatabaseConnection() { /* Open connection */ } ~DatabaseConnection() { /* Close connection */ } };
Conclusion
And there you have it, folks. Destructors might not be the sexiest part of programming, but they’re certainly one of the most important. By ensuring proper resource management, they help keep your programs running smoothly and efficiently. Whether you’re a seasoned developer or just starting out, understanding destructors is a key skill that will serve you well in your coding journey.
So, what are you waiting for? Start exploring destructors in your favorite programming language today. And don’t forget to share your thoughts in the comments below. Did I miss anything? Let me know, and I’ll be happy to help!
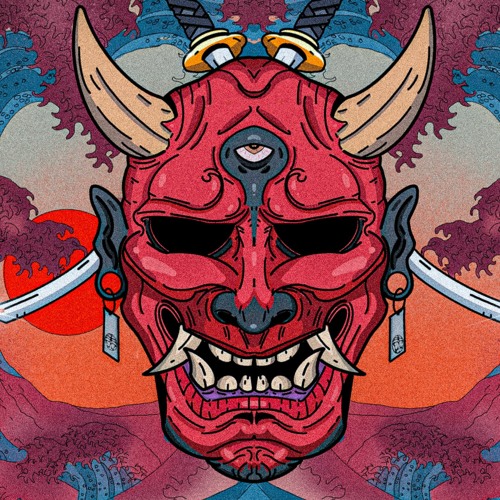
