Mastering Dart: The Ultimate Guide To Coding Like A Pro
Hey there, coding enthusiast! If you're reading this, chances are you're diving headfirst into the world of Dart programming. And trust me, you're in for an incredible ride. Dart is not just another programming language; it's a powerhouse that's taking the tech world by storm. Whether you're building web applications, mobile apps, or even server-side solutions, Dart has got your back. So, buckle up and let's explore everything you need to know about Dart!
Now, I know what you're thinking. "Why should I care about Dart when there are so many other languages out there?" Great question! Dart is special because it combines simplicity with incredible power. It's like having a Swiss Army knife for developers – versatile, efficient, and downright awesome. Plus, with Google backing it, you know it's here to stay.
Before we dive deep, let me give you a quick heads-up. This guide is packed with everything from the basics to advanced tips, so whether you're a beginner or a seasoned coder, you'll find something valuable here. And hey, if you're already a Dart pro, stick around – you might just learn a trick or two!
Read also:Johannes Lars The Rising Star In Modern Business Landscape
What is Dart Anyway?
Dart is a client-optimized programming language designed for fast apps on any platform. Yeah, that sounds fancy, but let's break it down. Dart was created by Google back in 2011, and it's been growing stronger ever since. It's like the younger, cooler cousin of JavaScript, but with a lot more muscle.
Here's the thing: Dart is all about speed and efficiency. It's built to handle everything from small scripts to large-scale applications. And with Flutter on its side, Dart has become the go-to choice for cross-platform app development.
Why Should You Learn Dart?
Let's face it: the coding world is competitive. But learning Dart can give you a serious edge. Here's why:
- Flutter Integration: Dart is the backbone of Flutter, one of the hottest frameworks for building mobile apps. If you're into app development, Dart is your golden ticket.
- Fast Compilation: Dart compiles super quickly, which means less waiting around and more coding time for you.
- Strong Community Support: With Google behind it, Dart has a thriving community and tons of resources to help you along the way.
Getting Started with Dart: The Basics
Alright, let's get our hands dirty. Before you can start building amazing apps, you'll need to understand the basics of Dart. Don't worry; it's not as scary as it sounds. Think of it like learning a new language – at first, it might seem tricky, but with a bit of practice, you'll be speaking fluently in no time.
Setting Up Your Environment
First things first: you'll need to set up your development environment. Here's what you need to do:
- Install the Dart SDK from the official website.
- Choose a code editor. VS Code with the Dart plugin is a popular choice.
- Create your first Dart file and give it a spin.
And just like that, you're ready to go. Easy, right?
Read also:Jerome James The Rising Star You Need To Know About
Understanding Dart Syntax
Now that you've got your environment set up, let's talk about Dart's syntax. It's clean, concise, and super easy to read. Here's a quick example:
dart
void main() {
print('Hello, Dart!');
}
See? That's all it takes to print "Hello, Dart!" to your console. Simple, isn't it? But don't let the simplicity fool you – Dart is packed with powerful features that make it a joy to work with.
Variables and Data Types
In Dart, you've got plenty of options when it comes to variables and data types. Here's a quick rundown:
- int: For whole numbers.
- double: For decimal numbers.
- String: For text.
- bool: For true or false values.
And the best part? Dart is type-safe, which means fewer bugs and more reliable code. Win-win!
Functions in Dart
Functions are the building blocks of any Dart program. They're like little machines that take inputs, do some work, and return outputs. Here's an example:
dart
void greet(String name) {
print('Hello, $name!');
}
Calling this function is as easy as:
dart
greet('Dart'); // Output: Hello, Dart!
See how that works? You can pass arguments to functions, and they'll do their thing. It's like magic, but with code.
Arrow Functions
For short functions, Dart has something called arrow functions. They're like the shorthand version of regular functions. Here's an example:
dart
int multiply(int a, int b) => a * b;
This is the same as:
dart
int multiply(int a, int b) {
return a * b;
}
Arrow functions are great for keeping your code clean and concise.
Classes and Objects
If you're familiar with object-oriented programming, you'll feel right at home with Dart. Classes and objects are the bread and butter of Dart development. Here's an example:
dart
class Person {
String name;
int age;
Person(this.name, this.age);
void introduce() {
print('Hi, my name is $name and I am $age years old.');
}
}
Creating an object is as simple as:
dart
var person = Person('John', 30);
person.introduce(); // Output: Hi, my name is John and I am 30 years old.
With classes and objects, you can create complex, reusable code structures that make your life as a developer much easier.
Inheritance in Dart
One of the coolest features of Dart is inheritance. It allows you to create new classes based on existing ones. Here's an example:
dart
class Student extends Person {
String school;
Student(String name, int age, this.school) : super(name, age);
void study() {
print('$name is studying at $school.');
}
}
Now you can create a Student object:
dart
var student = Student('Jane', 20, 'Dart University');
student.introduce(); // Output: Hi, my name is Jane and I am 20 years old.
student.study(); // Output: Jane is studying at Dart University.
Inheritance is a powerful tool that lets you build on existing code without reinventing the wheel.
Asynchronous Programming in Dart
In today's fast-paced world, asynchronous programming is a must. Dart makes it easy with the 'async' and 'await' keywords. Here's how it works:
dart
Future
await Future.delayed(Duration(seconds: 2));
return 'Data fetched successfully!';
}
void main() async {
String data = await fetchData();
print(data); // Output: Data fetched successfully!
}
With async and await, you can handle long-running tasks without blocking your program. It's like having a personal assistant to take care of the heavy lifting while you focus on other things.
Streams in Dart
Streams are another powerful feature of Dart. They allow you to handle events and data streams in real-time. Here's a simple example:
dart
Stream
for (int i = 1; i await Future.delayed(Duration(seconds: 1));
yield i;
}
}
void main() async {
await for (var i in countStream(5)) {
print(i);
}
}
Streams are perfect for handling things like user input, network data, and more. They keep your app responsive and dynamic.
Flutter and Dart: A Match Made in Heaven
When it comes to app development, Dart and Flutter are a dream team. Flutter uses Dart as its programming language, which means you can build beautiful, high-performance apps for both iOS and Android with just one codebase.
Here's a quick example of a Flutter widget:
dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter and Dart')),
body: Center(child: Text('Hello, Flutter!')),
),
);
}
}
With Flutter and Dart, the possibilities are endless. You can create anything from simple apps to complex, feature-rich applications.
Why Choose Flutter Over Other Frameworks?
There are plenty of app development frameworks out there, but Flutter stands out for several reasons:
- Hot Reload: See your changes instantly without restarting your app.
- Customizable Widgets: Flutter comes with a rich set of widgets that you can customize to your heart's content.
- Performance: Flutter apps are fast and smooth, thanks to Dart's efficient compilation.
So, if you're looking to build apps that impress, Flutter and Dart are the way to go.
Best Practices for Dart Developers
Now that you've got the basics down, let's talk about some best practices to help you become a Dart pro:
- Write Clean Code: Keep your code organized and easy to read. Trust me, your future self will thank you.
- Use Lint Rules: Dart has a set of lint rules that can help you catch potential issues before they become problems.
- Document Your Code: Comments are your friend. They make your code easier to understand and maintain.
Following these best practices will not only make you a better developer but also help you create code that's efficient, reliable, and easy to work with.
Tools and Resources for Dart Developers
Finally, let's talk about some tools and resources that can help you on your Dart journey:
- DartPad: An online editor where you can experiment with Dart code.
- Dart DevTools: A set of tools for debugging and profiling Dart applications.
- Dart Documentation: The official documentation is your go-to resource for everything Dart.
With these tools at your disposal, you'll be coding like a pro in no time.
Conclusion
And there you have it – your ultimate guide to mastering Dart. From the basics to advanced features, Dart has everything you need to become a coding powerhouse. So, what are you waiting for? Dive in and start building amazing apps!
Don't forget to share this article with your fellow coders, leave a comment, and check out our other guides. Together, let's make the world a better place, one line of code at a time!
Table of Contents
- What is Dart Anyway?
- Why Should You Learn Dart?
- Getting Started with Dart: The Basics
- Understanding Dart Syntax

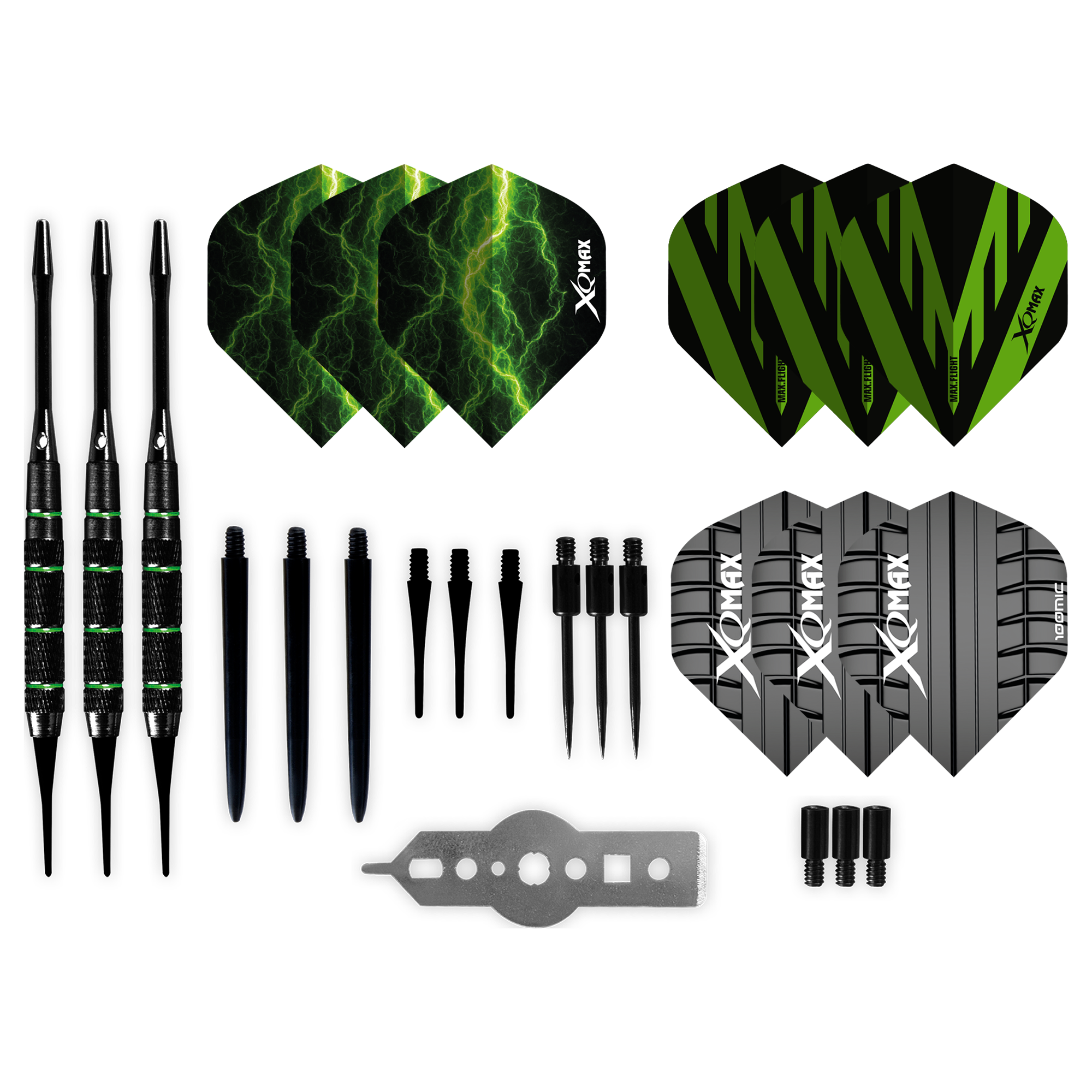
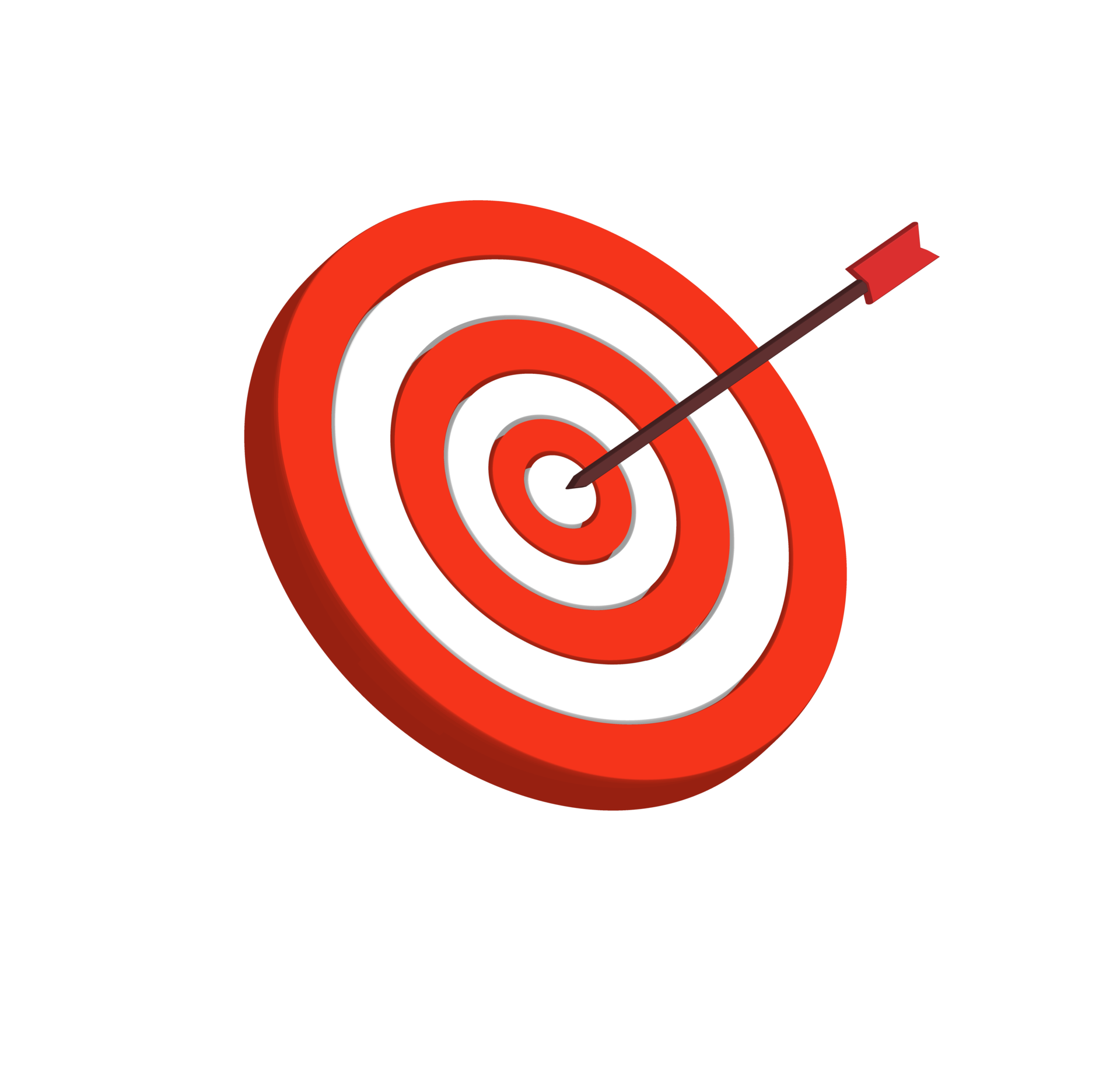